LibTorch
The DolphinDB LibTorch plugin allows users to directly load and use TorchScript models within the DolphinDB environment. This integration enables users to combine DolphinDB's powerful data processing capabilities with PyTorch's deep learning functionalities for efficient model prediction and data analysis.
Installation (with installPlugin
)
Required server version:
- Shark (DolphinDB GPU version) 3.00.1 or higher.
- CPU version: "LibTorch"
- GPU version: "GpuLibTorch" (CUDA 11.8 required).
- DolphinDB 3.00.2 or higher.
- CPU version: "LibTorch"
Supported OS: Linux x86-64.
Note:The current LibTorch plugin uses LibTorch version 2.1. To avoid compatibility issues, ensure that the model is also trained with LibTorch 2.1.
Installation Steps:
(1) Use listRemotePlugins
to check plugin information in the plugin repository.
Note: For plugins not included in the provided list, you can install through precompiled binaries or compile from source. These files can be accessed from our GitHub repository by switching to the appropriate version branch.
login("admin", "123456")
listRemotePlugins()
(2) Invoke installPlugin
for plugin installation. Use "LibTorch" for the CPU version and "GpuLibTorch" for the GPU version. Note that the two versions cannot be loaded at the same time.
installPlugin("LibTorch") // CPU version
installPlugin("GpuLibTorch") // GPU version
(3) Use loadPlugin
to load the plugin before using the plugin methods.
loadPlugin("LibTorch") // CPU version
loadPlugin("GpuLibTorch") // GPU version
Method References
load
Syntax
load(path)
Details
The method loads a PyTorch model and returns a handle. The handle will be automatically released when the session ends.
Parameters
- path: A STRING scalar indicating the path to the PyTorch model file to be loaded. Since the LibTorch library is used, the model file must be in TorchScript format.
Examples
model = LibTorch::load("path/model.pth")
predict
Syntax
predict(handle, input, [parseResult = true])
Details
The method uses input for prediction and returns the prediction result in DolphinDB format.
Parameters
- handle: The handle returned by the load interface.
- input: A tensor object or an array of tensor objects used as input data for prediction.
- parseResult (optional): Whether to parse the prediction result. The default value is
true
, which returns the result as a nested array. If set tofalse
, the result is returned in tensor format.
Return Value
The prediction result can be returned in either nested array format or tensor format.
When parseResult is true, the prediction result, up to 4 dimensions, is parsed and converted as follows:
PyTorch Prediction Result | DolphinDB |
---|---|
1D tensor | 1D array (vector) |
2D tensor | 2D nested array (vectors nested within a tuple) |
3D tensor | 3D nested array (vectors nested within 2 tuples) |
4D tensor | 4D nested array (vectors nested within 3 tuples) |
Examples
model = LibTorch::load("path/model.pth")
tensor1 = tensor([[0.7740, -1.7911], [0.0267, 0.1928]])
tensor2 = tensor([[0.0267, 0.1928], [-1.6544, 1.2538]])
ans = LibTorch::predict(model, [tensor1, tensor2])
setDevice
Syntax
LibTorch::setDevice(model, device, [deviceId])
Details
Specify the computing device for prediction. The deivce is “CPU” by default.
Parameters
- model: The handle returned by the load method.
- device: A STRING scalar indicating the computing device. It can be “CPU” or “CUDA”.
- deviceId (optional): An INT scalar indicating the CUDA device ID when parameter device is set to “CUDA”.
Examples
LibTorch::setDevice(model, "CUDA")
Tensor Conversion Rules
Data Form | DolphinDB Tensor |
---|---|
scalar | 1D tensor |
vector | 1D tensor |
matrix | 2D tensor |
table | 2D tensor |
tuple of vectors (each element is a vector of the same type) | 2D tensor |
tuple of matrices (each element is a matrix with the same dimensions and type) | 3D tensor |
tuple of tuples (each element is a tuple, and each element of the sub-tuples is a vector of the same type) | 3D tensor |
n-level nested tuple | n-D tensor (where n <= 10) |
Note:
(1) In PyTorch, a scalar is converted to a 0D tensor, whereas in DolphinDB, it is converted to a 1D tensor.
(2) The elements within a tensor must have the same data type.
(3) In DolphinDB, each element of a regular tuple of vector represents a column, for example:
t = [[1,2],[3,4],[5,6]]
tensor(t)
For the tuple [[1,2],[3,4],[5,6]]
where each vector represents a column, there are a total of two rows and three columns. The resulting tensor would be:
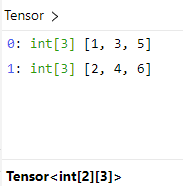
The setColumnarTuple!
function can be uesd to convert data into a row-based format similar to Python. For example:
t = [[1,2],[3,4],[5,6]].setColumnarTuple!()
tensor(t)
For the tuple [[1,2],[3,4],[5,6]]
where each vector represents a row, there are a total of three rows and two columns. The resulting tensor would be:
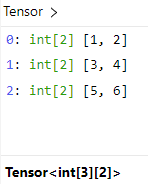
Similarly, if the input is a multi-dimensional nested array and it is desired to be converted to a tensor in a row-based format, the setColumnarTuple!
function can be used:
data = [[[1,2],[3,4],[5,6]], [[7,8],[9,10],[11,12]]]
for (i in 0: size(data)) {
data[i] = data[i].setColumnarTuple!()
}
tensor(data) // Converts to a tensor of shape 2 * 3 * 2
Usage Examples
loadPlugin("/path/PluginLibTorch.txt")
go
//Load data
data_vector = float(loadText("/path/closing_prices.csv").Closing_Price)
//The model prediction requires a 3D structure, so the input data needs preprocessing
//1. Load the data and normalize it
//2. Generate the data structure required for prediction using a sliding window of size 20
def prepareData(data_vector){
// Normalize the data to the range [-1, 1]
minv = min(data_vector)
maxv = max(data_vector)
data_normal = 2 * ((data_vector - minv) / (maxv - minv)) - 1
// Expand the data into a 3D structure with a sliding window of size 20
input = []
aggrJoin = defg(mutable re, data){
re.append!(matrix(data))
return 0
}
moving(aggrJoin{input}, data_normal, 20)
return input
}
// Preprocess the input dataset and convert the data to Tensor format
input = tensor(prepareData(data_vector))
// Load the model and make predictions
model = LibTorch::load("/path/scripted_model.pth")
output = LibTorch::predict(model, input)
// Denormalize the prediction results back to the original range
maxv = max(data_vector)
minv = min(data_vector)
result = (output + 1) * (maxv - minv) / 2 + minv