fixedLengthArrayVector
Syntax
fixedLengthArrayVector(args…)
Arguments
args can be vectors, tuples, fixed length array vectors, matrices, or tables. All args must be of the same data type supported by array vectors.
Details
Concatenate vectors, matrices, and tables and return an array vector.
Note: The length of a vector or each vector in a tuple, and the number of rows of a matrix or table must be the same.
The following figure describes how different data forms are concatenated into an array vector based on Example 1.
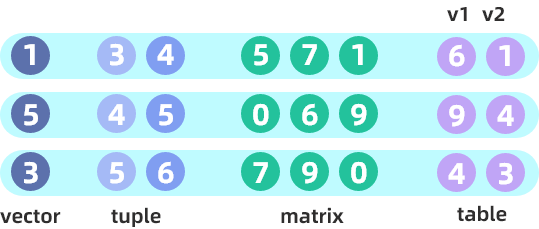
Examples
Example 1.
vec = 1 5 3
tp = [3 4 5, 4 5 6]
m = matrix(5 0 7, 7 6 9, 1 9 0)
tb = table(6 9 4 as v1, 1 4 3 as v2)
f = fixedLengthArrayVector(vec, tp, m, tb)
f;
// output: [[1,3,4,5,7,1,6,1],[5,4,5,0,6,9,9,4],[3,5,6,7,9,0,4,3]]
typestr(f);
// output: FAST INT[] VECTOR
Example 2. store multiple columns as one column
login("admin","123456")
syms="A"+string(1..30)
datetimes=2019.01.01T00:00:00..2019.01.31T23:59:59
n=200
if(existsDatabase("dfs://stock")) {
dropDatabase("dfs://stock")
}
db=database("dfs://stock", RANGE, cutPoints(syms,3), engine="TSDB");
t=table(take(datetimes,n) as trade_time, take(syms,n) as sym,take(500+rand(10.0,n), n) as bid1, take(500+rand(20.0,n),n) as bid2)
t1=select trade_time, sym, fixedLengthArrayVector(bid1,bid2) as bid from t
quotes=db.createPartitionedTable(t1,`quotes,`sym, sortColumns=`sym`trade_time).append!(t1)
select * from quotes
trade_time | sym | bid |
---|---|---|
2019.01.01T00:00:00 | A1 | [503.111142,507.55833] |
2019.01.01T00:00:30 | A1 | [502.991382,501.734092] |
2019.01.01T00:01:00 | A1 | [500.790709,509.200963] |
2019.01.01T00:01:30 | A1 | [501.127932,507.972508] |
2019.01.01T00:02:00 | A1 | [500.678614,514.947117] |
You can obtain a bid price by specifying the index. Applying a function to the bid column is equivalent to calculating on all bid prices.
select avg(bid[0]) as avg_bid1, avg(bid[1]) as avg_bid2, avg(bid) as avg_bid from quotes
avg_bid1 | avg_bid2 | avg_bid |
---|---|---|
505.0263 | 509.2912 | 507.16 |
Normally the field names of quotes are composed of the quote type and a number. To store multiple quote prices into an array vector, you can write the script as shown below:
// generate 50 bid/ask prices
n = 200
t=table(take(datetimes,n) as trade_time, take(syms,n) as sym)
for(i in 1:51){
t["bid"+string(i)] = take(500+rand(10.0,n), n)
}
// store the data into an array vector
t["bid"]=fixedLengthArrayVector(t["bid"+string(1..50)])
t1=select trade_time, sym, bid from t
To improve the performance, you can use it with function unifiedCall.
t["bid"]=unifiedCall(fixedLengthArrayVector, t["bid"+string(1..50)])
t1=select trade_time, sym, bid from t
// Generate 5 levels of quotes for exchanges A and B
syms = "A" + string(1..5)
datetimes = 2019.01.01T00:00:00..2019.01.31T23:59:59
n = 10
t1 = table(take(datetimes, n) as trade_time, take(syms, n) as sym)
for(i in 1:6){
t1["bid" + string(i)] = take(50 + rand(10.0, n), n)
}
t2 = table(take(datetimes, n) as trade_time, take(syms, n) as sym)
for(i in 1:6){
t2["bid" + string(i)] = take(50 + rand(10.0, n), n)
}
// save quotes as array vectors
t1["bid"] = fixedLengthArrayVector(t1["bid" + string(1..5)])
t2["bid"] = fixedLengthArrayVector(t2["bid" + string(1..5)])
// concatenate fixed length array vectors
t1["bid"] = fixedLengthArrayVector(t1["bid"], t2["bid"])
t3 = select trade_time, sym, bid from t1
trade_time | sym | bid |
---|---|---|
2019.01.01T00:00:00 | A1 | [56.68,54.55,53.11,53.38,59.60,57.35,59.92,50.62,56.06,54.69] |
2019.01.01T00:00:01 | A2 | [58.97,50.65,54.38,50.11,56.26,52.35,52.79,55.43,52.16,53.35] |
2019.01.01T00:00:02 | A3 | [50.25,53.45,52.68,58.19,56.51,57.54,55.22,51.74,58.63,57.43] |
2019.01.01T00:00:03 | A4 | [56.42,50.28,57.04,52.45,51.83,57.75,55.04,57.34,57.82,53.28] |
2019.01.01T00:00:04 | A5 | [59.90,51.73,55.54,57.74,53.48,59.62,57.26,53.99,52.67,57.82] |
2019.01.01T00:00:05 | A1 | [53.16,59.27,52.97,50.41,58.30,57.83,54.93,56.91,52.51,57.95] |
2019.01.01T00:00:06 | A2 | [53.14,50.87,52.62,54.47,59.97,56.99,55.32,54.66,56.77,58.39] |
2019.01.01T00:00:07 | A3 | [58.33,59.80,52.34,57.52,57.39,54.67,51.19,52.11,55.27,53.07] |
2019.01.01T00:00:08 | A4 | [55.21,54.88,54.38,52.36,56.56,53.81,57.84,53.24,54.87,54.63] |
2019.01.01T00:00:09 | A5 | [52.98,55.72,55.83,50.60,51.01,57.02,54.07,54.63,55.44,59.28] |